How can I create custom fields for taxonomies on WordPress? (PHP, Instruction 2023)
Marc Wagner
January 27, 2023
Just like for Post Types, you can also create custom fields for your WordPress taxonomies. The values can then be stored in the term metadata. How the whole thing works, I show you in this tutorial. I’ll also show you how to extend the admin table of your taxonomy to display your values directly. Finally, we add a sorting function.
Create, edit and save custom fields for WordPress taxonomies #
To extend your taxonomies, you need the following action hooks:
- Create Terms: {$taxonomy}_add_form_fields
- Edit Terms: {$taxonomy}_edit_form_fields
- Save terms: created_{$taxonomy} & edited_{$taxonomy}
{$taxonomy}_add_form_fields
First, we create an input for the field (we’ll call it “sort”). It is supposed to store a number that can be used to sort terms, for example. So that we can specify the field for our terms directly when we create them, we add the following PHP snippet:
/** * Taxonomie: book */ function add_form_field_to_taxonomy_book(string $taxonomy): void{ // add a nonce for security wp_nonce_field( 'book_meta_new', 'book_meta_new_nonce' ); ?> <tr class="form-field"> <th> <lable for="sort"><?php _e('Sort', 'my_domain'); ?></lable> </th> <td> <input name="sort" id="sort" type="number" value="<?php echo esc_attr($sort); ?>"/> <p class="description"><?php _e('Enter the position within the menu. Negativ values are allowed.', 'my_domain'); ?></p> </td> </tr> <?php } add_action('book_add_form_fields', 'add_form_field_to_taxonomy_book', 10, 1);
Now we have a field for entering the sort. Here’s an image of how it might look in your WordPress backend:
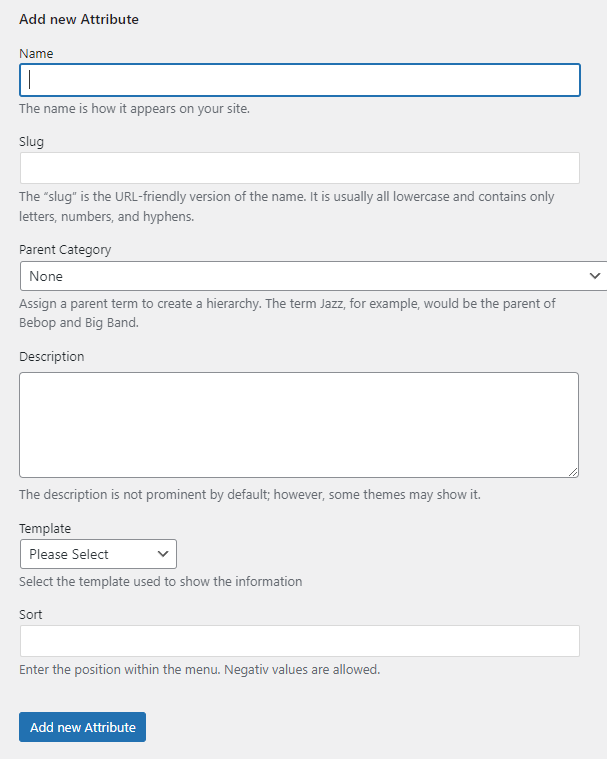
{$taxonomy}_edit_form_fields
Next, we need to make sure that we can edit the terms and thus our field. After all, we would certainly like to change the value at some point. In order for us to succeed, we need to add one more function:
public function edit_form_field_for_taxonomy_book(\WP_Term $term, string $taxonomy): void { $sort = get_term_meta($term->term_id, 'sort', true); // add a nonce for security wp_nonce_field( 'book_meta_edit', 'book_meta_edit_nonce' ); ?> <tr class="form-field"> <th> <label for="sort"><?php _e('Sort', 'f12_table'); ?></label> </th> <td> <input name="sort" id="sort" type="number" value="<?php echo esc_attr($sort); ?>"/> <p class="description"><?php _e('Enter the position within the menu. Negativ values are allowed.', 'f12_table'); ?></p> </td> </tr> <?php } add_action('book_edit_form_fields', 'edit_form_field_for_taxonomy_book', 10, 2);
After the installation, we are also finally shown a field for changing our value when editing the WordPress term. Here is an example of what this looked like in our case:
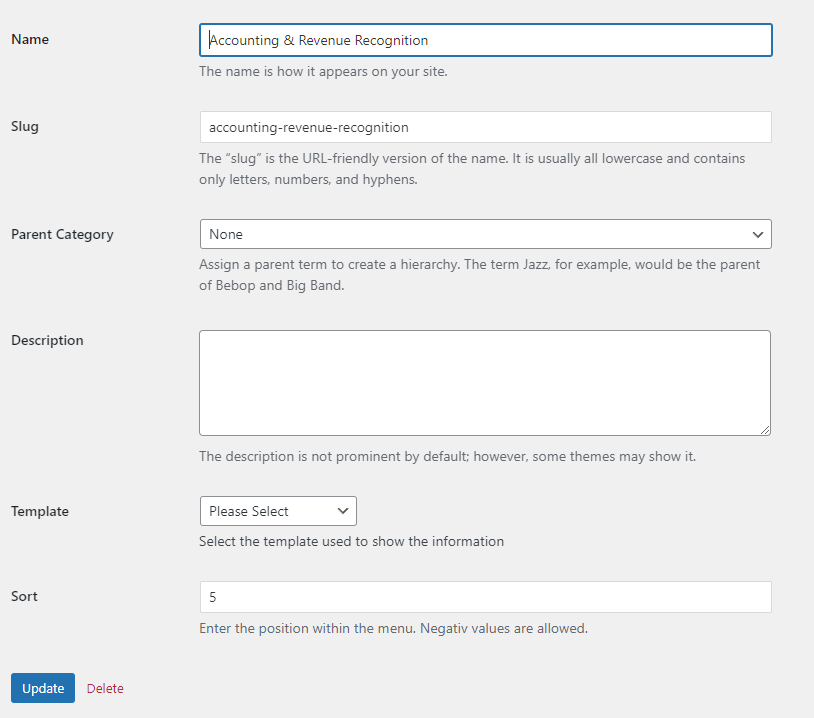
created_{$taxonomy} & edited_{$taxonomy}
Finally, of course, we need to make sure that our value is stored in the database. The following excerpt helps us here:
public function save(int $term_id) { if(!isset($_POST['sort'])){ return; } if(!isset($_POST['book_meta_new_nonce']) && !isset($_POST['book_meta_edit_nonce'])){ return; } if(!wp_verifiy_nonce($_POST['book_meta_new_nonce'],'book_meta_new') && !wp_verify_nonce($_POST['book_meta_edit_nonce'], 'book_meta_edit')){ return; } update_term_meta($term_id, 'sort', (int)($_POST['sort'])); } add_action('created_book', 'save_book_meta', 10, 1); add_action('edited_book', 'save_book_meta', 10, 1)
That’s it — we have completed all the steps to create a new field, edit it and save it to the database.
Output term metadata with a WordPress shortcode #
To output the data now, we create a shortcode. We can then place this on WordPress pages and posts as we see fit. Here is a PHP snippet to query a field from your term. It does not even have to be a term from the taxonomy ‘book’.
/** * Add shortcode [book_metafield term_id=123 meta_key="sort"] * @return string */ function render_book_metafield($args, $content = ''): string{ // parse the $args $args = shortcode_atts(['term_id' => 0,'meta_key' => 'sort'], $args, 'book_metafield'); $value = get_term_meta($args['term_id'], 'sort', true); if(!$value){ return 'Term not found'; } return value; } add_shortcode('book_metafield', 'render_book_metafield');
The call is then made like this: [book_metafield term_id=“123” meta_key=“sort”]
Extend the Taxonomy Admin table with a new column #
You can also expand the table of your taxonomy to display the value of the field directly in the overview. For this you need the following WordPress filter and action hooks:
- Add column: manage_edit-{$taxonomy}_columns
- Add content: manage_{$taxonomy}_custom_column
- Make sortable: manage_edit-{$taxonomy}_sortable_column
manage_edit-{$taxonomy}_columns
First, we tell WordPress that we want to display another column for our Taxonomy. For this we need to insert the following PHP snippet:
/** * Add new column to taxonomy book */ public function add_column_for_taxonomy_book(array $columns): array { $columns['sort'] = __('Sort', 'my_domain'); return $columns; } // Filter: manage_edit-{$taxonomy}_columns add_filter('manage_edit-book_columns', 'add_column_for_taxonomy_book', 10, 1)
manage_{$taxonomy}_custom_column
Now we need to expand the output of each line to show our value.
/** * Show content of term meta. */ public function add_content_for_taxonomy_book(string $content, string $column_name, int $term_id):void{ // Skip if this is not our column if($column_name != 'sort'){ return; } $sort = get_term_meta($term_id, 'sort', true); // Skip if term or field does not exist if(!$sort){ return; } echo esc_attr($sort); } // Action: manage_{$taxonomy}_custom_column add_action('manage_book_custom_column', 'add_content_for_taxonomy_book', 10, 3);
That’s about it. Now both the column and the row are output with our contents.

manage_edit-{$taxonomy}_sortable_columns
Finally, we extend the admin table so that we can sort by our field. For this we only need the following PHP snippet:
public function make_book_sortable(array $columns): array { // Add or remove columns here. If you remove them, it // will remove the sort function. $columns['sort'] = __('Sort', 'my_domain'); return $columns; } add_filter('manage_edit-book_sortable_columns', 'make_book_sortable', 10, 1);
After inserting, you can sort the terms by the new values in ascending and descending order by clicking on the column name.

Artikel von:
Marc Wagner
Hi Marc here. I’m the founder of Forge12 Interactive and have been passionate about building websites, online stores, applications and SaaS solutions for businesses for over 20 years. Before founding the company, I already worked in publicly listed companies and acquired all kinds of knowledge. Now I want to pass this knowledge on to my customers.